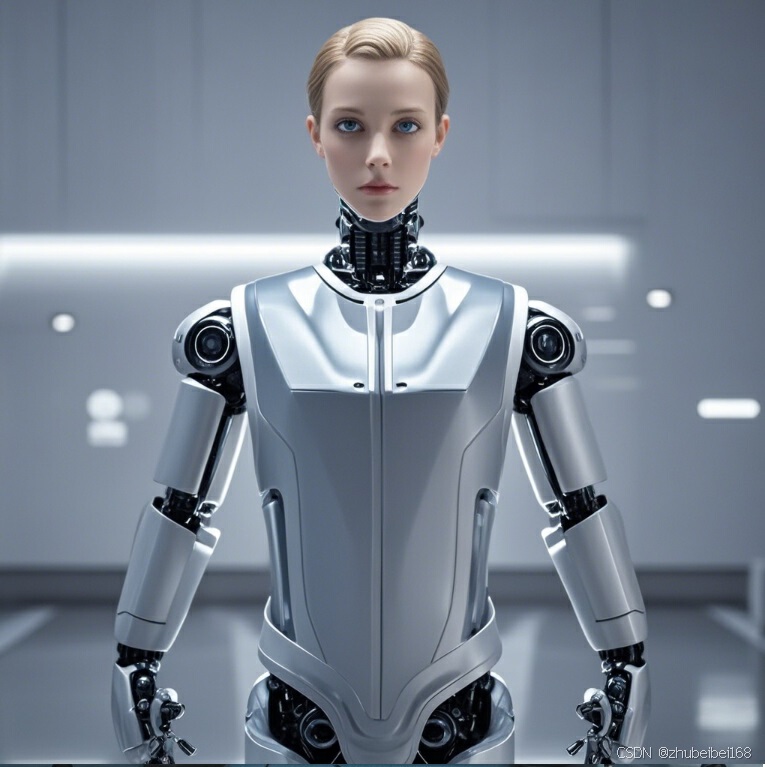
环境感知:障碍物检测_(6).深度学习在障碍物检测中的应用
选择合适的模型并进行定义。例如,使用 PyTorch 定义一个简单的 CNN 模型。深度学习在障碍物检测中的应用已经广泛应用于自动驾驶、机器人导航和安全监控等领域。通过不断优化模型架构、数据预处理方法和训练策略,我们可以进一步提升障碍物检测的性能,为实际应用提供更强大的技术支持。随着技术的不断进步,深度学习在障碍物检测中的应用前景将更加广阔。
深度学习在障碍物检测中的应用
在现代计算机视觉技术中,深度学习已经成为障碍物检测的重要手段。通过使用卷积神经网络(CNN)、循环神经网络(RNN)以及其他先进的深度学习模型,我们可以在各种复杂环境中准确地检测和识别障碍物。本节将详细介绍深度学习在障碍物检测中的应用,包括模型选择、数据预处理、训练过程、评估方法以及实际应用案例。
1. 模型选择
1.1 卷积神经网络(CNN)
卷积神经网络是深度学习中最常用的模型之一,尤其适用于图像处理任务。在障碍物检测中,CNN 通过卷积层、池化层和全连接层的组合,可以有效地提取图像中的特征,并进行分类和检测。
1.1.1 基本结构
-
卷积层:用于提取局部特征。
-
池化层:用于降低特征图的维度,减少计算量。
-
全连接层:用于将提取到的特征进行分类。
1.1.2 模型架构
常用的 CNN 架构包括:
-
AlexNet:早期的经典模型,奠定了 CNN 的基本框架。
-
VGGNet:通过增加网络深度来提高性能。
-
ResNet:通过残差连接解决了深度网络的梯度消失问题。
-
Inception:通过多尺度卷积提高了特征提取的效率。
1.2 目标检测模型
目标检测模型在障碍物检测中尤为重要,常见的目标检测模型包括:
-
R-CNN:使用Selective Search生成候选区域,然后通过 CNN 进行特征提取和分类。
-
Fast R-CNN:改进了 R-CNN,将候选区域生成和特征提取整合到一个网络中。
-
Faster R-CNN:进一步优化了 Fast R-CNN,使用区域提议网络(RPN)生成候选区域。
-
YOLO(You Only Look Once):将目标检测任务转化为一个回归问题,速度快且精度高。
-
SSD(Single Shot MultiBox Detector):在多个特征图上进行检测,适用于多种尺度的目标。
1.3 实例分割模型
实例分割不仅检测目标,还能精确地分割出目标的轮廓,适用于需要精确定位障碍物的场景。常用的实例分割模型包括:
-
Mask R-CNN:在 Faster R-CNN 的基础上增加了分割分支,实现了端到端的实例分割。
-
DETR(Detection Transformer):使用 Transformer 结构进行目标检测和分割,具有较好的性能。
2. 数据预处理
数据预处理是深度学习模型训练的重要步骤,包括图像增强、数据标准化和数据标注等。
2.1 图像增强
图像增强通过变换图像来增加数据的多样性,提高模型的泛化能力。常见的图像增强方法包括:
-
旋转:随机旋转图像。
-
缩放:随机缩放图像。
-
裁剪:随机裁剪图像。
-
翻转:水平或垂直翻转图像。
-
亮度调整:随机调整图像的亮度。
2.1.1 代码示例
使用 PyTorch 的 torchvision.transforms
进行图像增强:
import torchvision.transforms as transforms
from PIL import Image
# 定义图像增强的变换
transform = transforms.Compose([
transforms.RandomRotation(10), # 随机旋转10度
transforms.RandomResizedCrop(224), # 随机裁剪并缩放到224x224
transforms.RandomHorizontalFlip(), # 随机水平翻转
transforms.ColorJitter(brightness=0.2, contrast=0.2, saturation=0.2, hue=0.1), # 随机调整亮度、对比度、饱和度和色调
transforms.ToTensor(), # 转换为Tensor
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225]) # 标准化
])
# 读取图像
image = Image.open('path_to_image.jpg')
# 应用变换
augmented_image = transform(image)
# 显示增强后的图像
import matplotlib.pyplot as plt
plt.imshow(augmented_image.permute(1, 2, 0))
plt.show()
2.2 数据标准化
数据标准化是将图像数据转换为标准的数值范围,常用的方法是将像素值归一化到 [0, 1] 或进行 Z-score 标准化。
2.2.1 代码示例
使用 PyTorch 进行数据标准化:
import torch
import torchvision.transforms as transforms
from PIL import Image
# 读取图像
image = Image.open('path_to_image.jpg')
# 转换为Tensor
image_tensor = transforms.ToTensor()(image)
# 标准化
mean = torch.mean(image_tensor, dim=(1, 2))
std = torch.std(image_tensor, dim=(1, 2))
normalized_image = transforms.Normalize(mean=mean, std=std)(image_tensor)
# 显示标准化后的图像
plt.imshow(normalized_image.permute(1, 2, 0))
plt.show()
2.3 数据标注
数据标注是为图像中的每个障碍物提供边界框和类别标签。常见的标注工具包括 LabelMe、Labelbox 和 Supervisely。
2.3.1 标注工具
-
LabelMe:基于 Python 的开源标注工具,支持多边形标注。
-
Labelbox:在线标注平台,支持多种标注类型。
-
Supervisely:支持多种标注任务,包括实例分割和语义分割。
3. 训练过程
训练深度学习模型的过程包括数据加载、模型定义、损失函数选择、优化器配置和训练循环等。
3.1 数据加载
使用数据加载器将图像和标注数据加载到模型中。PyTorch 提供了 DataLoader
和 Dataset
类来帮助我们实现这一过程。
3.1.1 代码示例
定义一个自定义数据集类:
import os
import torch
from torch.utils.data import Dataset, DataLoader
from PIL import Image
import json
class ObstacleDataset(Dataset):
def __init__(self, root_dir, transform=None):
self.root_dir = root_dir
self.transform = transform
self.annotations = self.load_annotations()
def load_annotations(self):
# 读取标注文件
with open(os.path.join(self.root_dir, 'annotations.json'), 'r') as f:
annotations = json.load(f)
return annotations
def __len__(self):
return len(self.annotations)
def __getitem__(self, idx):
img_path = os.path.join(self.root_dir, self.annotations[idx]['filename'])
image = Image.open(img_path).convert('RGB')
labels = self.annotations[idx]['labels']
bboxes = self.annotations[idx]['bboxes']
if self.transform:
image = self.transform(image)
return image, labels, bboxes
# 创建数据集和数据加载器
dataset = ObstacleDataset(root_dir='path_to_dataset', transform=transform)
data_loader = DataLoader(dataset, batch_size=4, shuffle=True, num_workers=2)
3.2 模型定义
选择合适的模型并进行定义。例如,使用 PyTorch 定义一个简单的 CNN 模型。
3.2.1 代码示例
定义一个简单的 CNN 模型:
import torch
import torch.nn as nn
import torch.nn.functional as F
class SimpleCNN(nn.Module):
def __init__(self, num_classes):
super(SimpleCNN, self).__init__()
self.conv1 = nn.Conv2d(3, 16, kernel_size=3, stride=1, padding=1)
self.conv2 = nn.Conv2d(16, 32, kernel_size=3, stride=1, padding=1)
self.conv3 = nn.Conv2d(32, 64, kernel_size=3, stride=1, padding=1)
self.fc1 = nn.Linear(64 * 28 * 28, 512)
self.fc2 = nn.Linear(512, num_classes)
def forward(self, x):
x = F.relu(self.conv1(x))
x = F.max_pool2d(x, 2)
x = F.relu(self.conv2(x))
x = F.max_pool2d(x, 2)
x = F.relu(self.conv3(x))
x = F.max_pool2d(x, 2)
x = x.view(x.size(0), -1)
x = F.relu(self.fc1(x))
x = self.fc2(x)
return x
# 实例化模型
model = SimpleCNN(num_classes=10)
3.3 损失函数选择
选择合适的损失函数对模型的训练至关重要。常用的损失函数包括交叉熵损失(Cross-Entropy Loss)和均方误差损失(Mean Squared Error Loss)。
3.3.1 代码示例
使用交叉熵损失:
import torch.nn as nn
# 定义损失函数
criterion = nn.CrossEntropyLoss()
# 假设模型的输出和标签
outputs = model(images)
labels = torch.tensor([1, 2, 3, 4])
# 计算损失
loss = criterion(outputs, labels)
# 反向传播
loss.backward()
3.4 优化器配置
选择合适的优化器可以加速模型的收敛。常用的优化器包括 SGD、Adam 和 RMSprop。
3.4.1 代码示例
使用 Adam 优化器:
import torch.optim as optim
# 定义优化器
optimizer = optim.Adam(model.parameters(), lr=0.001)
# 优化器的更新步骤
optimizer.step()
3.5 训练循环
训练循环是模型训练的核心部分,包括前向传播、损失计算、反向传播和参数更新。
3.5.1 代码示例
完整的训练循环示例:
# 训练模型
num_epochs = 10
for epoch in range(num_epochs):
for images, labels, bboxes in data_loader:
# 前向传播
outputs = model(images)
# 计算损失
loss = criterion(outputs, labels)
# 反向传播和参数更新
optimizer.zero_grad()
loss.backward()
optimizer.step()
# 打印每个epoch的损失
print(f'Epoch [{epoch+1}/{num_epochs}], Loss: {loss.item():.4f}')
4. 评估方法
评估模型的性能是训练过程的重要环节。常用的评估指标包括准确率(Accuracy)、精确率(Precision)、召回率(Recall)和 F1 分数(F1 Score)。
4.1 准确率(Accuracy)
准确率是分类正确的样本数占总样本数的比例。
4.1.1 代码示例
计算准确率:
import torch
def accuracy(outputs, labels):
_, preds = torch.max(outputs, dim=1)
correct = (preds == labels).sum().item()
total = labels.size(0)
return correct / total
# 评估模型
model.eval()
with torch.no_grad():
for images, labels, bboxes in data_loader:
outputs = model(images)
acc = accuracy(outputs, labels)
print(f'Accuracy: {acc:.4f}')
4.2 精确率(Precision)
精确率是分类为正类的样本中实际为正类的样本比例。
4.3 召回率(Recall)
召回率是实际为正类的样本中分类为正类的样本比例。
4.4 F1 分数(F1 Score)
F1 分数是精确率和召回率的调和平均值,适用于不平衡数据集。
4.4.1 代码示例
使用 sklearn
计算 F1 分数:
from sklearn.metrics import f1_score
def f1_score_eval(outputs, labels):
_, preds = torch.max(outputs, dim=1)
preds = preds.cpu().numpy()
labels = labels.cpu().numpy()
return f1_score(labels, preds, average='weighted')
# 评估模型
model.eval()
with torch.no_grad():
for images, labels, bboxes in data_loader:
outputs = model(images)
f1 = f1_score_eval(outputs, labels)
print(f'F1 Score: {f1:.4f}')
5. 实际应用案例
5.1 自动驾驶
在自动驾驶中,障碍物检测是确保车辆安全的关键技术。通过使用深度学习模型,可以实时检测并分类前方的障碍物,如行人、车辆和路标。
5.1.1 代码示例
使用 Faster R-CNN 进行障碍物检测:
import torch
import torchvision
from torchvision.models.detection.faster_rcnn import FastRCNNPredictor
from torchvision.transforms import functional as F
from PIL import Image
import matplotlib.pyplot as plt
# 加载预训练的Faster R-CNN模型
model = torchvision.models.detection.fasterrcnn_resnet50_fpn(pretrained=True)
# 替换分类器以适应新的类别数
num_classes = 10 # 假设有10个类别
in_features = model.roi_heads.box_predictor.cls_score.in_features
model.roi_heads.box_predictor = FastRCNNPredictor(in_features, num_classes)
# 将模型设置为评估模式
model.eval()
# 读取图像
image = Image.open('path_to_image.jpg')
image = F.to_tensor(image).unsqueeze(0)
# 进行预测
with torch.no_grad():
predictions = model(image)
# 解析预测结果
pred_boxes = predictions[0]['boxes']
pred_labels = predictions[0]['labels']
pred_scores = predictions[0]['scores']
# 可视化预测结果
def plot_prediction(image, boxes, labels, scores, threshold=0.5):
image = image.squeeze(0).permute(1, 2, 0).cpu().numpy()
plt.imshow(image)
ax = plt.gca()
for box, label, score in zip(boxes, labels, scores):
if score > threshold:
x, y, x1, y1 = box
ax.add_patch(plt.Rectangle((x, y), x1 - x, y1 - y, fill=False, edgecolor='red', linewidth=1))
ax.text(x, y, f'{label} {score:.2f}', fontsize=12, color='white', verticalalignment='top', bbox={'facecolor': 'red', 'alpha': 0.5, 'pad': 2})
plt.show()
plot_prediction(image, pred_boxes, pred_labels, pred_scores)
5.2 机器人导航
在机器人导航中,障碍物检测用于路径规划和避障。通过实时检测环境中的障碍物,机器人可以安全地导航到目标位置。
5.2.1 代码示例
使用 YOLOv3 进行障碍物检测:
import torch
import torchvision
from torchvision.models.detection import yolo
from torchvision.transforms import functional as F
from PIL import Image
import matplotlib.pyplot as plt
# 加载预训练的YOLOv3模型
model = yolo.yolov3(pretrained=True)
# 替换分类器以适应新的类别数
num_classes = 10 # 假设有10个类别
in_features = model.classifier[3].in_features
model.classifier[3] = nn.Conv2d(in_features, num_classes, kernel_size=1)
# 将模型设置为评估模式
model.eval()
# 读取图像
image = Image.open('path_to_image.jpg')
image = F.to_tensor(image).unsqueeze(0)
# 进行预测
with torch.no_grad():
predictions = model(image)
# 解析预测结果
pred_boxes = predictions[0]['boxes']
pred_labels = predictions[0]['labels']
pred_scores = predictions[0]['scores']
# 可视化预测结果
def plot_prediction(image, boxes, labels, scores, threshold=0.5):
image = image.squeeze(0).permute(1, 2, 0).cpu().numpy()
plt.imshow(image)
ax = plt.gca()
for box, label, score in zip(boxes, labels, scores):
if score > threshold:
x, y, x1, y1 = box
ax.add_patch(plt.Rectangle((x, y), x1 - x, y1 - y, fill=False, edgecolor='red', linewidth=1))
ax.text(x, y, f'{label} {score:.2f}', fontsize=12, color='white', verticalalignment='top', bbox={'facecolor': 'red', 'alpha': 0.5, 'pad': 2})
plt.show()
plot_prediction(image, pred_boxes, pred_labels, pred_scores)
5.3 安全监控
在安全监控中,障碍物检测用于识别异常行为和潜在威胁。通过实时检测和分类环境中的障碍物,可以及时采取措施避免安全问题。
5.3.1 代码示例
使用 SSD 进行障碍物检测:
import torch
import torchvision
from torchvision.models.detection import ssd
from torchvision.transforms import functional as F
from PIL import Image
import matplotlib.pyplot as plt
# 加载预训练的SSD模型
model = ssd.ssd300_vgg16(pretrained=True)
# 替换分类器以适应新的类别数
num_classes = 10 # 假设有10个类别
in_features = model.classifier[3].in_features
model.classifier[3] = nn.Conv2d(in_features, num_classes, kernel_size=1)
# 将模型设置为评估模式
model.eval()
# 读取图像
image = Image.open('path_to_image.jpg')
image = F.to_tensor(image).unsqueeze(0)
# 进行预测
with torch.no_grad():
predictions = model(image)
# 解析预测结果
pred_boxes = predictions[0]['boxes']
pred_labels = predictions[0]['labels']
pred_scores = predictions[0]['scores']
# 可视化预测结果
def plot_prediction(image, boxes, labels, scores, threshold=0.5):
image = image.squeeze(0).permute(1, 2, 0).cpu().numpy()
plt.imshow(image)
ax = plt.gca()
for box, label, score in zip(boxes, labels, scores):
if score > threshold:
x, y, x1, y1 = box
ax.add_patch(plt.Rectangle((x, y), x1 - x, y1 - y, fill=False, edgecolor='red', linewidth=1))
ax.text(x, y, f'{label} {score:.2f}', fontsize=12, color='white', verticalalignment='top', bbox={'facecolor': 'red', 'alpha': 0.5, 'pad': 2})
plt.show()
plot_prediction(image, pred_boxes, pred_labels, pred_scores)
6. 结论
深度学习在障碍物检测中的应用已经取得了显著的进展。通过选择合适的模型、进行有效的数据预处理、配置优化的训练过程和采用合理的评估方法,可以在多种实际场景中实现高精度和高效率的障碍物检测。未来的研究方向包括进一步提高模型的实时性能、增强模型在复杂环境中的鲁棒性以及探索更多的应用场景。
6.1 未来研究方向
-
实时性能优化:通过模型剪枝、量化和硬件加速等技术,提高模型的实时性能,使其在嵌入式设备上也能高效运行。
-
鲁棒性增强:在更多的复杂场景和极端条件下进行训练和测试,增强模型的鲁棒性。
-
多模态融合:结合图像、激光雷达(LiDAR)、雷达等多种传感器的数据,提高障碍物检测的准确性和可靠性。
-
无监督和弱监督学习:探索无监督和弱监督学习方法,减少标注数据的依赖,降低训练成本。
-
迁移学习:利用预训练模型进行迁移学习,快速适应新的障碍物检测任务。
-
模型解释性:提高模型的解释性,使其在关键任务中更加可信和透明。
6.2 总结
深度学习在障碍物检测中的应用已经广泛应用于自动驾驶、机器人导航和安全监控等领域。通过不断优化模型架构、数据预处理方法和训练策略,我们可以进一步提升障碍物检测的性能,为实际应用提供更强大的技术支持。随着技术的不断进步,深度学习在障碍物检测中的应用前景将更加广阔。
更多推荐
所有评论(0)