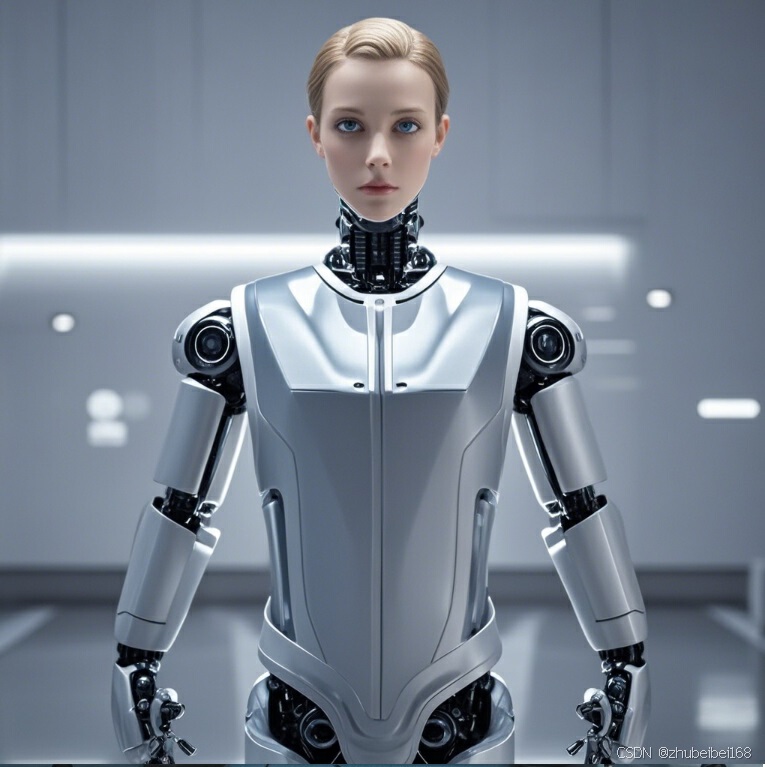
环境感知:障碍物检测_(2).障碍物检测技术概述
障碍物检测是环境感知中的一个核心问题,特别是在自动驾驶、机器人导航、无人机避障、安防监控和工业自动化等应用中,准确的障碍物检测对于系统的安全和可靠性至关重要。不同的传感器有不同的优缺点,因此在实际应用中往往需要结合多种传感器来提高检测的准确性和鲁棒性。常用的传感器包括摄像头、激光雷达(LIDAR)、毫米波雷达(RADAR)和超声波传感器等。常用的障碍物检测算法包括基于图像的边缘检测、深度学习方法、
障碍物检测技术概述
障碍物检测是环境感知中的一个核心问题,特别是在自动驾驶、机器人导航和无人机等应用中,准确的障碍物检测对于系统的安全和可靠性至关重要。本节将详细介绍障碍物检测的基本原理、常用技术以及应用场景。
1. 引言
障碍物检测是指通过传感器获取环境信息,进一步处理和分析这些信息以识别和定位环境中存在的障碍物。这些传感器可以包括摄像头、激光雷达(LIDAR)、毫米波雷达(RADAR)、超声波传感器等。不同的传感器有不同的优缺点,因此在实际应用中往往需要结合多种传感器来提高检测的准确性和鲁棒性。
2. 常用传感器及工作原理
2.1 摄像头
摄像头是最常见的视觉传感器,通过图像处理技术可以实现障碍物检测。摄像头获取的图像数据可以分为灰度图、RGB图、深度图等,不同类型的图像数据适合不同的处理方法。
2.1.1 图像预处理
图像预处理是障碍物检测的第一步,包括图像去噪、灰度化、边缘检测等。常见的预处理方法有高斯滤波、中值滤波等。
高斯滤波示例:
import cv2
import numpy as np
# 读取图像
image = cv2.imread('input_image.jpg')
# 高斯滤波
blurred_image = cv2.GaussianBlur(image, (5, 5), 0)
# 显示原始图像和处理后的图像
cv2.imshow('Original Image', image)
cv2.imshow('Blurred Image', blurred_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
2.2 激光雷达(LIDAR)
激光雷达通过发射激光并测量反射时间来获取环境的三维点云数据。点云数据可以用来构建环境的三维模型,进一步进行障碍物检测。
2.2.1 点云处理
点云处理通常包括点云滤波、聚类、分割等步骤。常用的点云处理库有PCL(Point Cloud Library)。
点云聚类示例:
import numpy as np
import open3d as o3d
# 读取点云数据
pcd = o3d.io.read_point_cloud('input_pcd.ply')
# 进行DBSCAN聚类
with o3d.utility.VerbosityContextManager(o3d.utility.VerbosityLevel.Debug) as cm:
labels = np.array(pcd.cluster_dbscan(eps=0.02, min_points=10))
# 绘制聚类结果
max_label = labels.max()
colors = plt.get_cmap("tab20")(labels / (max_label if max_label > 0 else 1))
colors[labels < 0] = 0 # 将噪声点设置为黑色
pcd.colors = o3d.utility.Vector3dVector(colors[:, :3])
o3d.visualization.draw_geometries([pcd])
2.3 毫米波雷达(RADAR)
毫米波雷达通过发射毫米波并接收反射波来获取障碍物的距离和速度信息。毫米波雷达在雨雪天气中表现较好,但分辨率较低。
2.3.1 信号处理
毫米波雷达的信号处理包括FFT(快速傅里叶变换)、CFAR(恒虚警率)等技术。
FFT示例:
import numpy as np
import matplotlib.pyplot as plt
# 生成模拟信号
fs = 1000 # 采样频率
t = np.arange(0, 1, 1/fs)
f = 5 # 信号频率
signal = np.sin(2 * np.pi * f * t)
# 进行FFT
fft_result = np.fft.fft(signal)
fft_freq = np.fft.fftfreq(t.shape[-1], 1/fs)
# 绘制FFT结果
plt.plot(fft_freq, np.abs(fft_result))
plt.xlabel('Frequency (Hz)')
plt.ylabel('Amplitude')
plt.title('FFT of the Signal')
plt.grid(True)
plt.show()
2.4 超声波传感器
超声波传感器通过发射超声波并接收反射波来测量障碍物的距离。超声波传感器成本低、使用简单,但检测范围有限。
2.4.1 距离测量
超声波传感器的距离测量基于声波的传播时间。
超声波传感器距离测量示例:
import RPi.GPIO as GPIO
import time
# 设置GPIO模式
GPIO.setmode(GPIO.BCM)
# 定义超声波传感器的引脚
TRIG = 23
ECHO = 24
# 设置引脚模式
GPIO.setup(TRIG, GPIO.OUT)
GPIO.setup(ECHO, GPIO.IN)
def measure_distance():
# 发射超声波
GPIO.output(TRIG, True)
time.sleep(0.00001)
GPIO.output(TRIG, False)
# 等待ECHO引脚变为高电平
while GPIO.input(ECHO) == 0:
pulse_start = time.time()
# 等待ECHO引脚变为低电平
while GPIO.input(ECHO) == 1:
pulse_end = time.time()
# 计算脉冲时间
pulse_duration = pulse_end - pulse_start
# 计算距离
distance = pulse_duration * 17150
distance = round(distance, 2)
return distance
# 测量距离
distance = measure_distance()
print(f"Distance: {distance} cm")
# 清理GPIO
GPIO.cleanup()
3. 常用障碍物检测算法
3.1 基于图像的障碍物检测
3.1.1 边缘检测
边缘检测是基于图像的障碍物检测中的一个重要步骤,常用的边缘检测算法有Canny、Sobel等。
Canny边缘检测示例:
import cv2
import numpy as np
# 读取图像
image = cv2.imread('input_image.jpg', cv2.IMREAD_GRAYSCALE)
# Canny边缘检测
edges = cv2.Canny(image, 100, 200)
# 显示边缘图像
cv2.imshow('Edges', edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
3.1.2 深度学习方法
深度学习方法在障碍物检测中表现出色,常用的网络模型有YOLO、Faster R-CNN等。
YOLO障碍物检测示例:
import cv2
import numpy as np
# 加载YOLO模型
net = cv2.dnn.readNetFromDarknet('yolov3.cfg', 'yolov3.weights')
layer_names = net.getLayerNames()
output_layers = [layer_names[i[0] - 1] for i in net.getUnconnectedOutLayers()]
# 读取图像
image = cv2.imread('input_image.jpg')
height, width, channels = image.shape
# 进行前向传播
blob = cv2.dnn.blobFromImage(image, 0.00392, (416, 416), (0, 0, 0), True, crop=False)
net.setInput(blob)
outs = net.forward(output_layers)
# 解析检测结果
class_ids = []
confidences = []
boxes = []
for out in outs:
for detection in out:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5:
# 获取物体的中心坐标和宽度高度
center_x = int(detection[0] * width)
center_y = int(detection[1] * height)
w = int(detection[2] * width)
h = int(detection[3] * height)
# 计算物体的边界框
x = int(center_x - w / 2)
y = int(center_y - h / 2)
boxes.append([x, y, w, h])
confidences.append(float(confidence))
class_ids.append(class_id)
# 非极大值抑制
indexes = cv2.dnn.NMSBoxes(boxes, confidences, 0.5, 0.4)
# 绘制检测结果
font = cv2.FONT_HERSHEY_PLAIN
colors = np.random.uniform(0, 255, size=(len(classes), 3))
if len(indexes) > 0:
for i in indexes.flatten():
x, y, w, h = boxes[i]
label = str(classes[class_ids[i]])
confidence = confidences[i]
color = colors[i]
cv2.rectangle(image, (x, y), (x + w, y + h), color, 2)
cv2.putText(image, f'{label} {confidence:.2f}', (x, y + 30), font, 3, color, 3)
# 显示检测结果
cv2.imshow('Image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
3.2 基于点云的障碍物检测
3.2.1 RANSAC算法
RANSAC(随机抽样一致性)算法常用于点云数据的平面拟合,从而识别地面和其他障碍物。
RANSAC平面拟合示例:
import numpy as np
import open3d as o3d
# 读取点云数据
pcd = o3d.io.read_point_cloud('input_pcd.ply')
# RANSAC平面拟合
plane_model, inliers = pcd.segment_plane(distance_threshold=0.01, ransac_n=3, num_iterations=1000)
[a, b, c, d] = plane_model
print(f"Plane equation: {a:.2f}x + {b:.2f}y + {c:.2f}z + {d:.2f} = 0")
# 提取地面点和障碍物点
inlier_cloud = pcd.select_by_index(inliers)
outlier_cloud = pcd.select_by_index(inliers, invert=True)
# 绘制结果
inlier_cloud.paint_uniform_color([0.5, 0.5, 0.5])
outlier_cloud.paint_uniform_color([1, 0, 0])
o3d.visualization.draw_geometries([inlier_cloud, outlier_cloud])
3.2.2 Voxel Grid滤波
Voxel Grid滤波将点云数据划分为一个个小的体素,然后对每个体素内的点进行处理,以减少点云数据的密度。
Voxel Grid滤波示例:
import open3d as o3d
# 读取点云数据
pcd = o3d.io.read_point_cloud('input_pcd.ply')
# Voxel Grid滤波
voxel_pcd = pcd.voxel_down_sample(voxel_size=0.05)
# 绘制结果
o3d.visualization.draw_geometries([voxel_pcd])
3.3 融合多种传感器数据
融合多种传感器数据可以提高障碍物检测的准确性和鲁棒性。常见的融合方法有多传感器数据融合(MSDF)、多传感器信息融合(MIF)等。
3.3.1 传感器数据融合
传感器数据融合通常包括数据对齐、数据融合、结果融合等步骤。
数据对齐示例:
import numpy as np
import cv2
# 读取图像和点云数据
image = cv2.imread('input_image.jpg')
pcd = o3d.io.read_point_cloud('input_pcd.ply')
# 点云数据投影到图像平面
intrinsic = o3d.camera.PinholeCameraIntrinsic(
o3d.camera.PinholeCameraIntrinsicParameters.PrimeSenseDefault)
extrinsic = np.eye(4) # 假设外部参数为单位矩阵
depth = np.asarray(pcd.points)[:, 2].reshape((480, 640))
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
image = o3d.geometry.Image(image)
rgbd_image = o3d.geometry.RGBDImage.create_from_color_and_depth(image, o3d.geometry.Image(depth), convert_rgb_to_intensity=False)
# 投影点云到图像
pcd_projected = o3d.geometry.PointCloud.create_from_rgbd_image(rgbd_image, intrinsic, extrinsic)
# 绘制结果
o3d.visualization.draw_geometries([pcd_projected])
4. 障碍物检测的应用场景
4.1 自动驾驶
在自动驾驶中,障碍物检测是确保车辆安全行驶的关键技术。通过摄像头、激光雷达等传感器获取的环境信息,可以实时检测前方的行人、车辆等障碍物。
自动驾驶障碍物检测示例:
import cv2
import numpy as np
# 加载YOLO模型
net = cv2.dnn.readNetFromDarknet('yolov3.cfg', 'yolov3.weights')
layer_names = net.getLayerNames()
output_layers = [layer_names[i[0] - 1] for i in net.getUnconnectedOutLayers()]
# 读取图像
image = cv2.imread('input_image.jpg')
height, width, channels = image.shape
# 进行前向传播
blob = cv2.dnn.blobFromImage(image, 0.00392, (416, 416), (0, 0, 0), True, crop=False)
net.setInput(blob)
outs = net.forward(output_layers)
# 解析检测结果
class_ids = []
confidences = []
boxes = []
for out in outs:
for detection in out:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5 and class_id in [2, 7]: # 2表示汽车,7表示行人
# 获取物体的中心坐标和宽度高度
center_x = int(detection[0] * width)
center_y = int(detection[1] * height)
w = int(detection[2] * width)
h = int(detection[3] * height)
# 计算物体的边界框
x = int(center_x - w / 2)
y = int(center_y - h / 2)
boxes.append([x, y, w, h])
confidences.append(float(confidence))
class_ids.append(class_id)
# 非极大值抑制
indexes = cv2.dnn.NMSBoxes(boxes, confidences, 0.5, 0.4)
# 绘制检测结果
font = cv2.FONT_HERSHEY_PLAIN
colors = np.random.uniform(0, 255, size=(len(classes), 3))
if len(indexes) > 0:
for i in indexes.flatten():
x, y, w, h = boxes[i]
label = str(classes[class_ids[i]])
confidence = confidences[i]
color = colors[i]
cv2.rectangle(image, (x, y), (x + w, y + h), color, 2)
cv2.putText(image, f'{label} {confidence:.2f}', (x, y + 30), font, 3, color, 3)
# 显示检测结果
cv2.imshow('Image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
4.2 机器人导航
在机器人导航中,障碍物检测用于规划路径、避免碰撞等。通过激光雷达和摄像头的组合,可以实现实时的环境感知。
机器人导航障碍物检测示例:
import cv2
import numpy as np
import open3d as o3d
# 读取图像
image = cv2.imread('input_image.jpg')
# 高斯滤波
blurred_image = cv2.GaussianBlur(image, (5, 5), 0)
# Canny边缘检测
edges = cv2.Canny(blurred_image, 100, 200)
# 读取点云数据
pcd = o3d.io.read_point_cloud('input_pcd.ply')
# RANSAC平面拟合
plane_model, inliers = pcd.segment_plane(distance_threshold=0.01, ransac_n=3, num_iterations=1000)
[a, b, c, d] = plane_model
print(f"Plane equation: {a:.2f}x + {b:.2f}y + {c:.2f}z + {d:.2f} = 0")
# 提取地面点和障碍物点
inlier_cloud = pcd.select_by_index(inliers)
outlier_cloud = pcd.select_by_index(inliers, invert=True)
# 绘制结果
inlier_cloud.paint_uniform_color([0.5, 0.5, 0.5])
outlier_cloud.paint_uniform_color([1, 0, 0])
o3d.visualization.draw_geometries([inlier_cloud, outlier_cloud])
# 显示边缘图像
cv2.imshow('Edges', edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
4.3 无人机避障
无人机避障通过摄像头和激光雷达等传感器实时检测前方的障碍物,并进行路径规划。无人机避障的难点在于高速飞行时的实时性要求。为了确保无人机的安全性和可靠性,通常会结合多种传感器的数据进行综合处理。
无人机避障示例:
import cv2
import numpy as np
# 加载YOLO模型
net = cv2.dnn.readNetFromDarknet('yolov3.cfg', 'yolov3.weights')
layer_names = net.getLayerNames()
output_layers = [layer_names[i[0] - 1] for i in net.getUnconnectedOutLayers()]
# 读取图像
image = cv2.imread('input_image.jpg')
height, width, channels = image.shape
# 进行前向传播
blob = cv2.dnn.blobFromImage(image, 0.00392, (416, 416), (0, 0, 0), True, crop=False)
net.setInput(blob)
outs = net.forward(output_layers)
# 解析检测结果
class_ids = []
confidences = []
boxes = []
for out in outs:
for detection in out:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5 and class_id in [2, 7, 14]: # 2表示汽车,7表示行人,14表示其他障碍物
# 获取物体的中心坐标和宽度高度
center_x = int(detection[0] * width)
center_y = int(detection[1] * height)
w = int(detection[2] * width)
h = int(detection[3] * height)
# 计算物体的边界框
x = int(center_x - w / 2)
y = int(center_y - h / 2)
boxes.append([x, y, w, h])
confidences.append(float(confidence))
class_ids.append(class_id)
# 非极大值抑制
indexes = cv2.dnn.NMSBoxes(boxes, confidences, 0.5, 0.4)
# 绘制检测结果
font = cv2.FONT_HERSHEY_PLAIN
colors = np.random.uniform(0, 255, size=(len(classes), 3))
if len(indexes) > 0:
for i in indexes.flatten():
x, y, w, h = boxes[i]
label = str(classes[class_ids[i]])
confidence = confidences[i]
color = colors[i]
cv2.rectangle(image, (x, y), (x + w, y + h), color, 2)
cv2.putText(image, f'{label} {confidence:.2f}', (x, y + 30), font, 3, color, 3)
# 显示检测结果
cv2.imshow('Image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
4.4 安防监控
在安防监控中,障碍物检测可以用于识别异常行为和入侵者。通过摄像头和传感器的组合,可以实时监控环境并及时发出警报。
安防监控障碍物检测示例:
import cv2
import numpy as np
# 读取视频流
cap = cv2.VideoCapture('input_video.mp4')
# 初始化背景减除器
fgbg = cv2.createBackgroundSubtractorMOG2()
while cap.isOpened():
ret, frame = cap.read()
if not ret:
break
# 应用背景减除
fgmask = fgbg.apply(frame)
# 阈值处理
_, thresh = cv2.threshold(fgmask, 250, 255, cv2.THRESH_BINARY)
# 形态学操作
kernel = cv2.getStructuringElement(cv2.MORPH_ELLIPSE, (5, 5))
opening = cv2.morphologyEx(thresh, cv2.MORPH_OPEN, kernel)
# 轮廓检测
contours, _ = cv2.findContours(opening, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
for contour in contours:
if cv2.contourArea(contour) < 500:
continue
(x, y, w, h) = cv2.boundingRect(contour)
cv2.rectangle(frame, (x, y), (x + w, y + h), (0, 255, 0), 2)
# 显示结果
cv2.imshow('Frame', frame)
cv2.imshow('Mask', opening)
if cv2.waitKey(30) & 0xFF == ord('q'):
break
# 释放资源
cap.release()
cv2.destroyAllWindows()
4.5 工业自动化
在工业自动化中,障碍物检测可以用于监控生产线上的物品位置和状态,确保机器人的安全操作。通过摄像头和激光雷达等传感器,可以实时检测生产线上的障碍物。
工业自动化障碍物检测示例:
import cv2
import numpy as np
import open3d as o3d
# 读取图像
image = cv2.imread('input_image.jpg')
# 高斯滤波
blurred_image = cv2.GaussianBlur(image, (5, 5), 0)
# Canny边缘检测
edges = cv2.Canny(blurred_image, 100, 200)
# 读取点云数据
pcd = o3d.io.read_point_cloud('input_pcd.ply')
# Voxel Grid滤波
voxel_pcd = pcd.voxel_down_sample(voxel_size=0.05)
# RANSAC平面拟合
plane_model, inliers = voxel_pcd.segment_plane(distance_threshold=0.01, ransac_n=3, num_iterations=1000)
[a, b, c, d] = plane_model
print(f"Plane equation: {a:.2f}x + {b:.2f}y + {c:.2f}z + {d:.2f} = 0")
# 提取地面点和障碍物点
inlier_cloud = voxel_pcd.select_by_index(inliers)
outlier_cloud = voxel_pcd.select_by_index(inliers, invert=True)
# 绘制结果
inlier_cloud.paint_uniform_color([0.5, 0.5, 0.5])
outlier_cloud.paint_uniform_color([1, 0, 0])
o3d.visualization.draw_geometries([inlier_cloud, outlier_cloud])
# 显示边缘图像
cv2.imshow('Edges', edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
5. 总结
障碍物检测是环境感知中的一个核心问题,特别是在自动驾驶、机器人导航、无人机避障、安防监控和工业自动化等应用中,准确的障碍物检测对于系统的安全和可靠性至关重要。不同的传感器有不同的优缺点,因此在实际应用中往往需要结合多种传感器来提高检测的准确性和鲁棒性。常用的传感器包括摄像头、激光雷达(LIDAR)、毫米波雷达(RADAR)和超声波传感器等。常用的障碍物检测算法包括基于图像的边缘检测、深度学习方法、基于点云的RANSAC算法和Voxel Grid滤波等。通过这些技术和算法,可以有效地实现障碍物的检测和识别,从而为各种应用提供可靠的支持。
更多推荐
所有评论(0)